Blog
Learn Python – Basic Day 2
- October 22, 2023
- Posted by: Saiful Bhuiyan
- Category: AI Premium Contents Class Assignment
No Comments
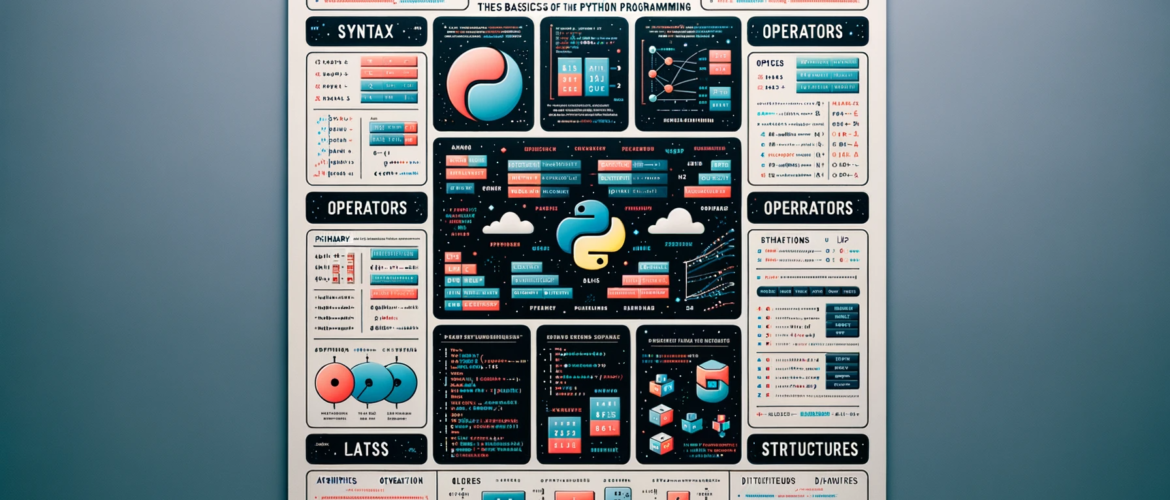
Python Syntax
- Indentation: Python uses indentation to define blocks of code. A block of code is a group of statements that should be treated as a unit.
- Example:
if 5 > 2: print("Five is greater than two!") - Comments: Comments are used to explain the code and make it more readable. Python ignores comments when running the code.
- Example:
# This is a comment print("Hello, World!")
Python Operators
- Arithmetic Operators: Arithmetic operators are used to perform arithmetic operations like addition, subtraction, multiplication, and division.
- Example:
x = 5 y = 2 print(x + y) print(x - y) print(x * y) print(x / y) - Comparison Operators: Comparison operators are used to compare two values.
- Example:
x = 5 y = 2 print(x == y) print(x != y) print(x > y) print(x < y) print(x >= y) print(x <= y)
Python Data Structures
- Lists: A list is a collection of items that are ordered and changeable.
- Example:
thislist = ["apple", "banana", "cherry"] print(thislist) - Tuples: A tuple is a collection of items that are ordered and unchangeable.
- Example:
thistuple = ("apple", "banana", "cherry") print(thistuple) - Dictionaries: A dictionary is a collection of items that are unordered, changeable, and indexed by keys.
- Example:
thisdict = { "brand": "Ford", "model": "Mustang", "year": 1964 } print(thisdict)
Interactive Session:
- Questions and topics for discussion:
- What are the different types of operators in Python?
- What are the different data structures in Python?
- Can you think of any real-life situations where you would use lists, tuples, or dictionaries?
Quiz:
- Questions and Answers:
-
What is indentation in Python?
- A) The amount of space at the beginning of a line of code
- B) The amount of space at the end of a line of code
- C) The amount of space between two lines of code
- Explanation: Indentation is the amount of space at the beginning of a line of code. Python uses indentation to define blocks of code. For example, in the code
if 5 > 2: print("Five is greater than two!")
, theprint
statement is indented to show that it is part of theif
block.
-
What are comments in Python?
- A) Comments are used to explain the code and make it more readable*
- B) Comments are used to make the code run faster
- C) Comments are used to make the code run slower
- Explanation: Comments are used to explain the code and make it more readable. Python ignores comments when running the code. For example, in the code
# This is a comment print("Hello, World!")
, the# This is a comment
is a comment and is ignored by Python.
-
What are the different types of operators in Python?
- A) Arithmetic operators and comparison operators*
- B) Arithmetic operators and logical operators
- C) Comparison operators and logical operators
- Explanation: There are several types of operators in Python, including arithmetic operators (e.g.,
+
,-
,*
,/
), comparison operators (e.g.,==
,!=
,>
,<
,>=
,<=
), and logical operators (e.g.,and
,or
,not
).
-
What are the different data structures in Python?
- A) Lists, tuples, and dictionaries*
- B) Lists, arrays, and dictionaries
- C) Arrays, tuples, and dictionaries
- Explanation: Python has several built-in data structures, including lists, tuples, and dictionaries. A list is a collection of items that are ordered and changeable. A tuple is a collection of items that are ordered and unchangeable. A dictionary is a collection of items that are unordered, changeable, and indexed by keys.
-
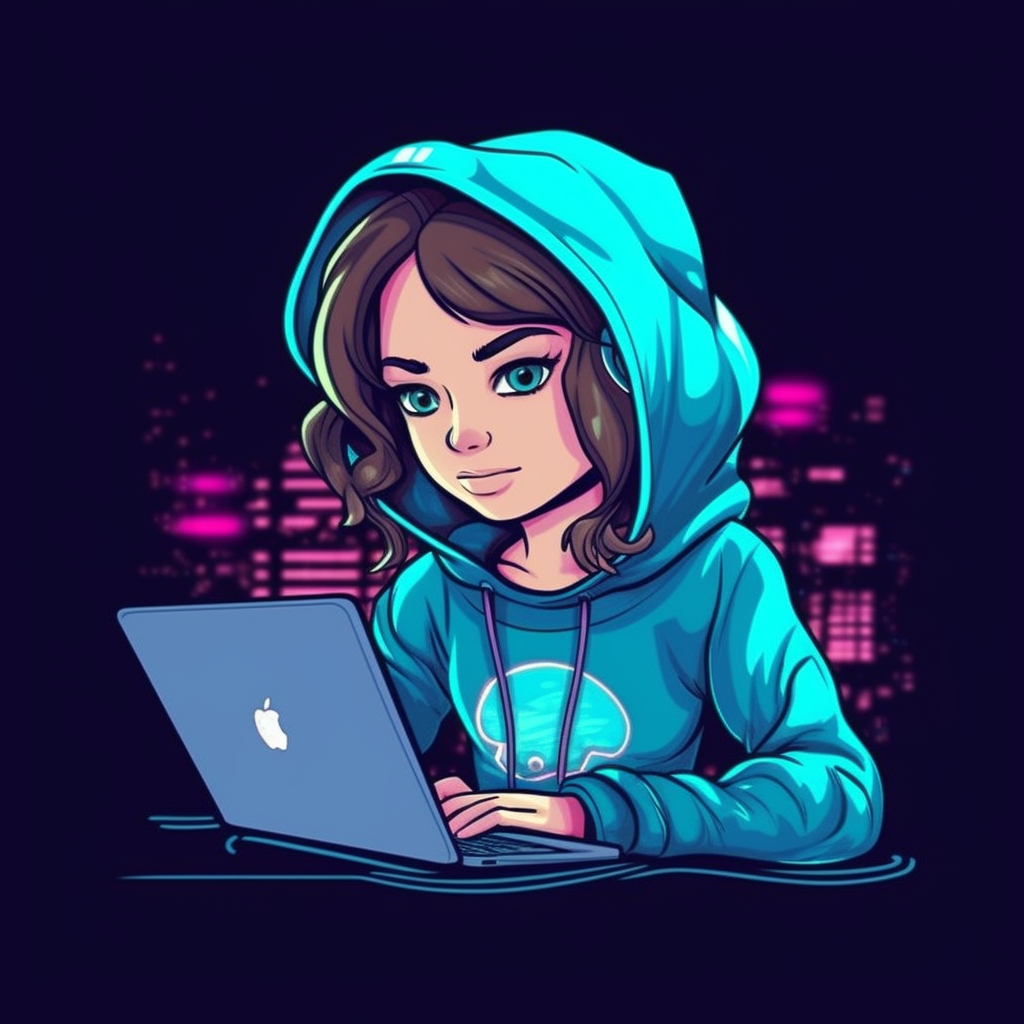
Mini-Project: Shopping List
- Objective: Create a program that allows the user to create and modify a shopping list.
- Requirements:
- The user should be able to add items to the shopping list.
- The user should be able to remove items from the shopping list.
- The user should be able to view the shopping list.
- Steps to Complete the Project:
- Write the code to display the options to the user (add item, remove item, view list, exit).
- Write the code to add an item to the shopping list.
- Write the code to remove an item from the shopping list.
- Write the code to view the shopping list.
- Source Code:
# Step 1: Create an empty shopping list
shopping_list = []
# Step 2: Display the options to the user
while True:
print("\nOptions:")
print("1. Add item")
print("2. Remove item")
print("3. View list")
print("4. Exit")
option = int(input("Enter an option (1-4): "))
# Step 3: Add item to the shopping list
if option == 1:
item = input("Enter the item to add: ")
shopping_list.append(item)
# Step 4: Remove item from the shopping list
elif option == 2:
item = input("Enter the item to remove: ")
if item in shopping_list:
shopping_list.remove(item)
else:
print("Item not in list")
# Step 5: View the shopping list
elif option == 3:
print("Shopping List:")
for item in shopping_list:
print(item)
# Step 6: Exit the program
elif option == 4:
break
else:
print("Invalid option. Please try again.")
In this code:
- Step 1: We create an empty shopping list.
- Step 2: We display the options to the user and get their input.
- Step 3: We add an item to the shopping list.
- Step 4: We remove an item from the shopping list.
- Step 5: We view the shopping list.
- Step 6: We exit the program.
Leave a Reply Cancel reply
You must be logged in to post a comment.